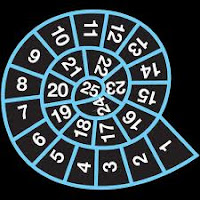
1) C Code to rotate a matrix by 90 degree to the right.
#include<stdio.h>
int main()
{
int i, j , n , a[10][10] , b[10][10];
printf("enter the number one less that the order of matrix\n");
scanf ("%d",&n);
printf ("enter the nxn matrix\n");
for (i=0;i<=n;i++)
for (j=0;j<=n;j++)
{
scanf("%d",&a[i][j]);
b[j][n-i]=a[i][j];
}
i=0;
printf ("before rotation: \n");
while (i<=n)
{
for (j=0;j<=n;j++)
printf("%d \t",a[i][j]);
printf("\n");
i++;
}
printf ("\n");
i=0;
printf ("after rotation: \n");
while (i<=n)
{
for (j=0;j<=n;j++)
{
printf("%d \t",b[i][j]);
}
printf("\n");
i++;
}
}
int main()
{
int i, j , n , a[10][10] , b[10][10];
printf("enter the number one less that the order of matrix\n");
scanf ("%d",&n);
printf ("enter the nxn matrix\n");
for (i=0;i<=n;i++)
for (j=0;j<=n;j++)
{
scanf("%d",&a[i][j]);
b[j][n-i]=a[i][j];
}
i=0;
printf ("before rotation: \n");
while (i<=n)
{
for (j=0;j<=n;j++)
printf("%d \t",a[i][j]);
printf("\n");
i++;
}
printf ("\n");
i=0;
printf ("after rotation: \n");
while (i<=n)
{
for (j=0;j<=n;j++)
{
printf("%d \t",b[i][j]);
}
printf("\n");
i++;
}
}
2) C code for spiral matrix
//Program to print a spiral matrix
#include <stdio.h>
#define max 1000
int p ,i,a[max][max],k=1,n,j;
int main()
{
int h=0;
printf ("enter a order of spiral matrix");
scanf ("%d",&p);
n=p;
i=j=1;
while (1)
{
if (i== 1+h && j== 1+h)
{
while (j!=p-h+1)
{
a[i][j] = k;
j++;
k++;
}
printf ("\n");
j--;
i++;
}
if (j==n && i!=n+1)
{
while (i!=n+1)
{
a[i][j] = k;
i++;
k++;
}
i=i-1;
}
if (j==n && i==n)
{
j--;
while (j!=h)
{
a[i][j] = k;
k++;
j--;
}
j=j+1;
}
if (i==n && j==h+1 )
{
i--;
while (i !=h+1 )
{
a[i][j] = k;
k++;
i--;
}
i++;
j++;
}
if (k>=p*p+1)
break;
n--;
h++;
}
for(i=1;i<=p;i++)
{
for(j=1;j<=p;j++)
printf ("%d", a[i][j]);
printf ("\n");
}
}
3) C Code for pattern-matching:
//program for pattern matching; order(m*n) time algorithm and space-complexity order(m+n)
#include <stdio.h>
int main ()
{
char a[500],b[200] , c , d;
int r=0,s=0 , i=0,k=0,j=0,p=0;
printf ("enter the text:\n");
while (1)
{
c=getchar();
if (c!='\n')
{
a[i]=c;
i++;
r++;
}
else break;
}
for (i=0;i<r;i++)
printf ("%c",a[i]);
printf ("\n");
i=0;
printf ("enter the pattern\n");
while (1)
{
d=getchar();
if (d!='\n')
{
b[i]=d;
i++;
s++;
}
else break;
}
for (i=0;i<s;i++)
printf ("%c",b[i]);
printf("\n");
i=0;
while (k!=r)
{
while (j!=s)
{
if (a[i]==b[j])
{
i++;
j++;
if (j==s)
p++;
}
else break;
}
j=0;
k++;
i=k;
}
printf ("no. of matches of pattern with text :%d\n",p);
}
#define max 1000
int p ,i,a[max][max],k=1,n,j;
int main()
{
int h=0;
printf ("enter a order of spiral matrix");
scanf ("%d",&p);
n=p;
i=j=1;
while (1)
{
if (i== 1+h && j== 1+h)
{
while (j!=p-h+1)
{
a[i][j] = k;
j++;
k++;
}
printf ("\n");
j--;
i++;
}
if (j==n && i!=n+1)
{
while (i!=n+1)
{
a[i][j] = k;
i++;
k++;
}
i=i-1;
}
if (j==n && i==n)
{
j--;
while (j!=h)
{
a[i][j] = k;
k++;
j--;
}
j=j+1;
}
if (i==n && j==h+1 )
{
i--;
while (i !=h+1 )
{
a[i][j] = k;
k++;
i--;
}
i++;
j++;
}
if (k>=p*p+1)
break;
n--;
h++;
}
for(i=1;i<=p;i++)
{
for(j=1;j<=p;j++)
printf ("%d", a[i][j]);
printf ("\n");
}
}
3) C Code for pattern-matching:
//program for pattern matching; order(m*n) time algorithm and space-complexity order(m+n)
#include <stdio.h>
int main ()
{
char a[500],b[200] , c , d;
int r=0,s=0 , i=0,k=0,j=0,p=0;
printf ("enter the text:\n");
while (1)
{
c=getchar();
if (c!='\n')
{
a[i]=c;
i++;
r++;
}
else break;
}
for (i=0;i<r;i++)
printf ("%c",a[i]);
printf ("\n");
i=0;
printf ("enter the pattern\n");
while (1)
{
d=getchar();
if (d!='\n')
{
b[i]=d;
i++;
s++;
}
else break;
}
for (i=0;i<s;i++)
printf ("%c",b[i]);
printf("\n");
i=0;
while (k!=r)
{
while (j!=s)
{
if (a[i]==b[j])
{
i++;
j++;
if (j==s)
p++;
}
else break;
}
j=0;
k++;
i=k;
}
printf ("no. of matches of pattern with text :%d\n",p);
}
No comments:
Post a Comment